How to Implement Routing in React JS?
Hello Coders, Today I am Demonastare Routing in React JS using Bootstrap So first we need to understand what is Routing? Basically, Routing is the process of moving one page to another page.
Project Name: Routing Project using React JS
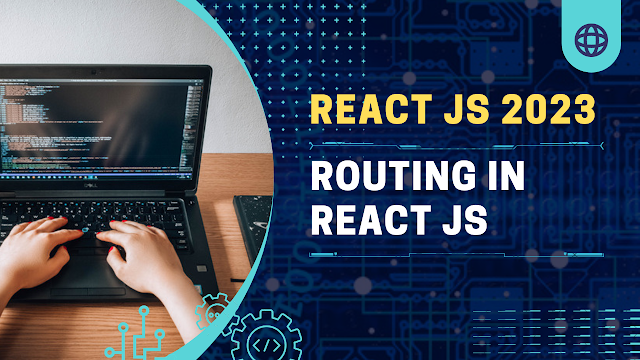
-------------------------------------------------------------------------
Step1: Create a new React JS project using npx command:
npx create-react-app my-routing-app
cd my-routing-app
-------------------------------------------------------------------
Step2: In React JS we need to install react-router using the npm command:
npm install react-router-dom
--------------------------------------------------------------------
Step3: Install bootstarp in React JS
In this project, we created one simple navigation bar using Bootstrap so first we need to install Bootstrap in our project.
npm install bootstrap
Also, we need to install JQuery without jQuery bootstrap is not working completely as expected:
npm install jquery
After installing Bootstrap and JQuery now we can go into our codebase. Just Click in file explore and click cmd
Code .
After entering this command visual stdio code editer is open in your terminal.
First We need to start our React JS project:
Step4: npm start
---------------------------------------------------------------------
After hitting this command React Project is running by default in the localhost:3000 port.
First, we need to import our bootstrap and JQuery file in the index.js page. After adding this file in React JS. Just add a simple line of code for the button like the primary button code your bootsrap is working or not.
import React, { StrictMode } from 'react';
import { createRoot } from 'react-dom/client';
import $ from 'jquery';
import Popper from 'popper.js';
import 'bootstrap/dist/css/bootstrap.min.css';
import 'bootstrap/dist/js/bootstrap.bundle.min';
import App from './App';
const root = createRoot(document.getElementById('app'));
root.render(
<StrictMode>
<App />
</StrictMode>
);
Please add this code in the App.js file:
<button type="button" className="btn btn-primary">Primary Button</button>
import React from 'react';
import './style.css';
export default function App() {
return (
<div>
<h1>Hello</h1>
<button type="button" className="btn btn-primary">
Primary Button
</button>
</div>
);
}
After adding this code to your app.js file just check in the browser if the blue button is visible or not. If yes then your bootstrap is successfully installed on your project.
------------------------------------------------------------------------------
Step 5:
Please create one component folder and create Navbar.js, Home.js, About.js, Service.js, Gallery.js, and Contact.js components in React JS.
Navbar.js:
-------------------------------------------------------------
import React from 'react'
function Navbar() {
return (
<div>Navbar</div>
)
}
export default Navbar
--------------------------------------------------------------
Home.js:
Just add a few lines of code like the above:
import React from 'react'
function Home() {
return (
<div>Home</div>
)
}
export default Home
----------------------------------
About.js
Just create functional components like the above:
import React from 'react'
function About() {
return (
<div>About</div>
)
}
export default About
-------------------------------------
Just create a Service component like this:
Service.js
import React from 'react'
function Service() {
return (
<div>Service</div>
)
}
export default Service
---------------------------------------------
Create one more component like
Gallery.js and written the following code snippet
import React from 'react'
function Gallery() {
return (
<div>Gallery</div>
)
}
export default Gallery
----------------------------------------
Contact.js: Add functional code like the above component:
import React from 'react'
function Contact() {
return (
<div>Contact</div>
)
}
export default Contact
-----------------------------------------------------------
Now we need to add the navbar code in the navbar component.
Navbar.js
--------------
in return, we need to write the following code:
-----------------------------------------------------------------------
import React from 'react';
import './Navbar.css';
function Navbar() {
return (
<>
<nav className="navbar navbar-expand-lg bg-body-tertiary navbar-custome">
<div className="container-fluid">
<a className="navbar-brand navbar-font-color" href="#">
Logo
</a>
<button
className="navbar-toggler"
type="button"
data-bs-toggle="collapse"
data-bs-target="#navbarNavDropdown"
aria-controls="navbarNavDropdown"
aria-expanded="false"
aria-label="Toggle navigation"
>
<span className="navbar-toggler-icon"></span>
</button>
<div className="collapse navbar-collapse" id="navbarNavDropdown">
<ul className="navbar-nav">
<li className="nav-item">
<a
className="nav-link navbar-font-color"
aria-current="page"
href="#"
>
Home
</a>
</li>
<li className="nav-item">
<a className="nav-link navbar-font-color" href="#">
About us
</a>
</li>
<li className="nav-item">
<a className="nav-link navbar-font-color" href="#">
Service
</a>
</li>
<li className="nav-item">
<a className="nav-link navbar-font-color" href="#">
Gallery
</a>
</li>
<li className="nav-item">
<a className="nav-link navbar-font-color" href="#">
Contact
</a>
</li>
</ul>
</div>
</div>
</nav>
</>
);
}
export default Navbar;
--------------------------------------------------
In this Navbar I am adding a few lines of CSS like this:
.navbar-color {
background-color: brown !important;
}
.nav-c-underline {
text-decoration: none !important;
color: white;
}
-------------------------------------------------------------
After adding this code see the following output are you showing:
Now we write the React Routing Code:
React Routing component:
The Main Components of React Router are:
- BrowserRouter: BrowserRouter is a router implementation that uses the HTML5 history API(pushState, replaceState, and the popstate event) to keep your UI in sync with the URL. It is the parent component that is used to store all of the other components.
- Routes: It’s a new component introduced in the v6 and an upgrade of the component. The main advantages of Routes over Switch are:
- Relative s and s
- Routes are chosen based on the best match instead of being traversed in order.
- Route: Route is the conditionally shown component that renders some UI when its path matches the current URL.
- Link: The link component is used to create links to different routes and implement navigation around the application. It works like an HTML anchor tag.
Now we need to wrap up the navbar link in the link tag.
Navbar.js
------------
import React from 'react';
import './Navbar.css';
import { Link } from 'react-router-dom';
function Navbar() {
return (
<>
<nav className="navbar navbar-expand-lg bg-body-tertiary navbar-color">
<div className="container-fluid">
<a className="navbar-brand nav-c-underline" href="#">
Logo
</a>
<button
className="navbar-toggler"
type="button"
data-bs-toggle="collapse"
data-bs-target="#navbarNavDropdown"
aria-controls="navbarNavDropdown"
aria-expanded="false"
aria-label="Toggle navigation"
>
<span className="navbar-toggler-icon"></span>
</button>
<div className="collapse navbar-collapse" id="navbarNavDropdown">
<ul className="navbar-nav">
<li className="nav-item">
<a className="nav-link" aria-current="page">
<Link to="/" className="nav-c-underline">
Home
</Link>
</a>
</li>
<li className="nav-item">
<a className="nav-link">
<Link to="/about" className="nav-c-underline">
About Us
</Link>
</a>
</li>
<li className="nav-item">
<a className="nav-link">
<Link to="/service" className="nav-c-underline">
Service
</Link>
</a>
</li>
<li className="nav-item">
<a className="nav-link">
<Link to="/gallery" className="nav-c-underline">
Gallery
</Link>
</a>
</li>
<li className="nav-item">
<a className="nav-link">
<Link to="/contact" className="nav-c-underline">
Contact
</Link>
</a>
</li>
</ul>
</div>
</div>
</nav>
</>
);
}
export default Navbar;
In the app.js file, we need to wrap the Router
and exact path-matching routing:
import React from 'react';
import './style.css';
import Navbar from './components/Navbar';
import Home from './components/Home';
import About from './components/About';
import Service from './components/Service';
import Gallery from './components/Gallery';
import Contact from './components/Contact';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
export default function App() {
return (
<Router>
<div>
<Navbar />
<Routes>
<Route exact path="/" element={<Home />}></Route>
<Route exact path="/about" element={<About />}></Route>
<Route exact path="/service" element={<Service />}></Route>
<Route exact path="/gallery" element={<Gallery />}></Route>
<Route exact path="/contact" element={<Contact />}></Route>
</Routes>
</div>
</Router>
);
}
Finally, our routing is implemented and it is working as expected.
Check Live Demo: Click Here
No comments:
Post a Comment